micro:bit and the PCF8591
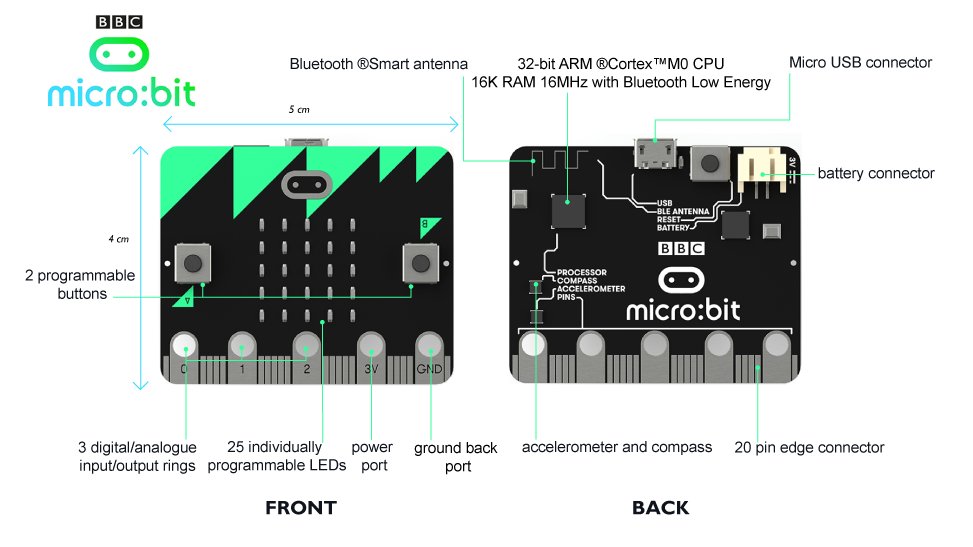
Shopping around for some components I came upon this module.
It is based upon the PCF8591 chip which contains 4 analogue inputs and one analogue output and has the following sensors on board to play with:
- Light sensor
- Thermistor
- Potentiometer
This module can be communicated with over i2c and is a relatively cheap board to play with.
Connecting to the micro:bit
As the module uses i2c it's simple to connect to the micro:bit.
- Pin 19 on the micro:bit connects to the SCL connection
- Pin 20 on the micro:bit connects to the SDA connection
- 3V connects to the power connection
- GND pin to to Ground
To make this easier it may be wise to use one of the available third party break out boards.
Communicating
Using mu, the following code snippet will read the value of the light sensor and display it on the micro:bit display.
from microbit import *
import struct
i2c.write(0x48,struct.pack('<hh',0x00))
while True:
output=i2c.read(0x48,5)
output=i2c.read(0x48,5)
display.scroll(struct.unpack('B',output)[0])
sleep(2000)
Explanation
i2c.write(0x48,struct.pack('<hh',0x00))
Writes the value 0x00 to the module at the address 0x48. This instructs the chip to read value at the analogue input 0. Changing this value from 0x00 to 0x03 will select the relevant input channel. Setting it to 0x04 will enable auto-increment meaning that on each read the next input will be selected.
output=i2c.read(0x48,5)
Requests five bytes from the selected input on the module. This is repeated twice as the actual analogue to digital conversion is carried out during the request but the previous conversion value is returned.